Hello Dear Students. In Today’s article, I am going to teach you how to create a basic calculator that can perform arithmetic operations like addition, subtraction, multiplication, and division. we are going to build a simple calculator using Python programming language. if you are a beginner and have started to learn Python then this small Python project will help you to learn Python basics with practice.
Define Arithmetic Functions
First of all, we create some functions for performing arithmetic operations. To define a function in Python we use the “def” keyword. if you want to create any function with any name you need to add the “def” keyword just before the name of the function
def add(x, y):
return x + y
The above code will create a function. Using that function we can add two numbers. like if x=2,y=2 then the function will return 4…
let’s define some more functions.
def add(x, y):
"""Function to add two numbers"""
return x + y
def subtract(x, y):
"""Function to subtract two numbers"""
return x - y
def multiply(x, y):
"""Function to multiply two numbers"""
return x * y
def divide(x, y):
"""Function to divide two numbers"""
if y == 0:
return "Error! Division by zero."
else:
return x / y
In this above code, we’re creating four functions: add, subtract, multiply, and divide.
add
: This function takes two numbers, x and y, and returns their sum.subtract
: This function takes two numbers,x
andy
, and returns their difference.multiply
: This function takes two numbers,x
andy
, and returns their product.divide
: This function takes two numbers,x
andy
, and returns their quotient. But it checks if the second numbery
is zero because we can’t divide by zero. Ify
is zero, it shows an error message. Otherwise, it is dividedx
byy
and returns the result.
Display Menu and Get User Input
As we want to perform some arithmetic operations before that we need to print all the operation names on the screen. if we want to print anything using Python we need to use the “print’ function.
print("Select operation:")
print("1. Addition")
print("2. Subtraction")
print("3. Multiplication")
print("4. Division")
Here, we’re printing a menu for the user to see the available arithmetic operations: addition, subtraction, multiplication, and division.
Perform Selected Operation
As I know you are a beginner so don’t worry I will explain everything step by step. This part is where the actual calculations happen. we are using the “while” keyword here to create a loop.
while True:
choice = input("Enter choice (1/2/3/4): ")
if choice in ('1', '2', '3', '4'):
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print("Result:", add(num1, num2))
elif choice == '2':
print("Result:", subtract(num1, num2))
elif choice == '3':
print("Result:", multiply(num1, num2))
elif choice == '4':
print("Result:", divide(num1, num2))
break
else:
print("Invalid input. Please enter a valid number (1/2/3/4).")
let me give a brief about all the functions used in the above code.
- We use a
while
loop to keep asking the user for input until they provide a valid choice. - We get the user’s choice of operation and store it in the variable
choice
. - We then check if the choice is one of the valid options (1, 2, 3, or 4).
- If the choice is valid, we ask the user to enter two numbers,
num1
andnum2
, for the calculation. - Based on the choice, we call the corresponding function (
add
,subtract
,multiply
, ordivide
) withnum1
andnum2
as arguments and print the result. - If the user enters an invalid choice, we print an error message and ask them to enter a valid choice.
Code Testing
def add(x, y):
"""Function to add two numbers"""
return x + y
def subtract(x, y):
"""Function to subtract two numbers"""
return x - y
def multiply(x, y):
"""Function to multiply two numbers"""
return x * y
def divide(x, y):
"""Function to divide two numbers"""
if y == 0:
return "Error! Division by zero."
else:
return x / y
print("Select operation:")
print("1. Addition")
print("2. Subtraction")
print("3. Multiplication")
print("4. Division")
while True:
choice = input("Enter choice (1/2/3/4): ")
if choice in ('1', '2', '3', '4'):
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print("Result:", add(num1, num2))
elif choice == '2':
print("Result:", subtract(num1, num2))
elif choice == '3':
print("Result:", multiply(num1, num2))
elif choice == '4':
print("Result:", divide(num1, num2))
break
else:
print("Invalid input. Please enter a valid number (1/2/3/4).")
Output:

Performing addition operation.
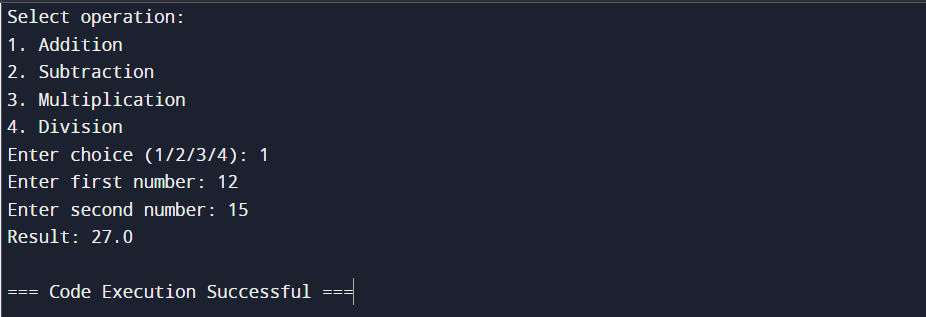
Performing Subtract operation.
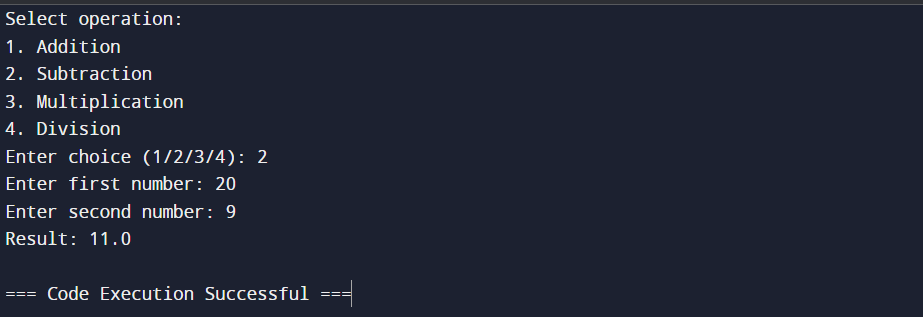
Performing Multiply operation

Performing Division operation
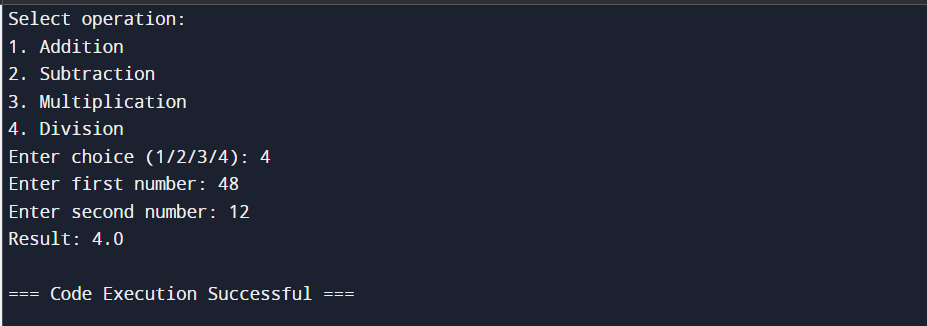
Congrats you have successfully created a simple calculator or a mini Python project.