Table of Contents
Java program to check if a number is an Armstrong number. An Armstrong number (also known as a narcissistic number or pluperfect number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because 13+53+33=1531^3 + 5^3 + 3^3 = 15313+53+33=153.
Here’s a Java program that checks if a given number is an Armstrong number.
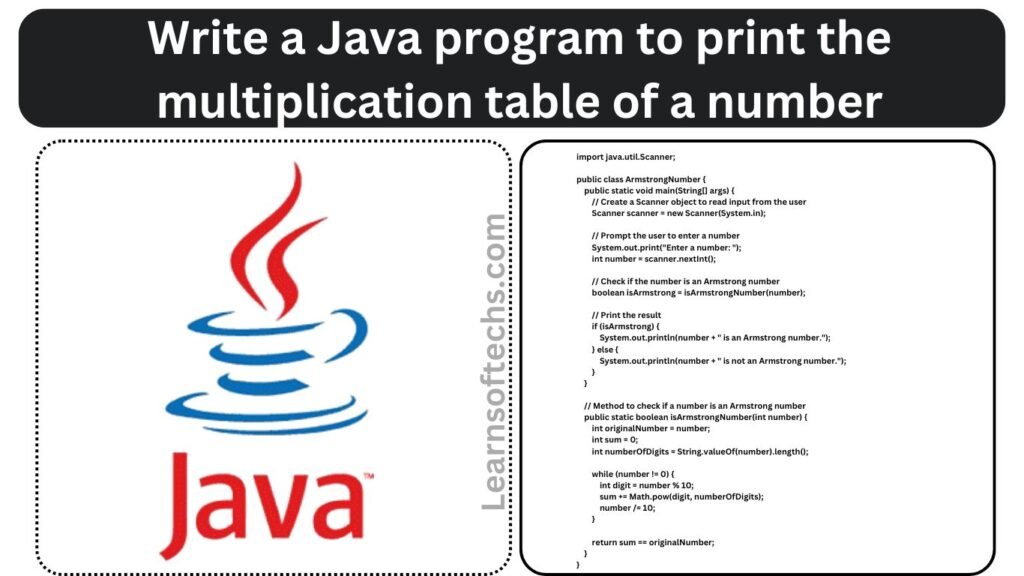
Java Program to Check if a Number is an Armstrong Number
import java.util.Scanner;
public class ArmstrongNumber {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter a number
System.out.print("Enter a number: ");
int number = scanner.nextInt();
// Check if the number is an Armstrong number
boolean isArmstrong = isArmstrongNumber(number);
// Print the result
if (isArmstrong) {
System.out.println(number + " is an Armstrong number.");
} else {
System.out.println(number + " is not an Armstrong number.");
}
}
// Method to check if a number is an Armstrong number
public static boolean isArmstrongNumber(int number) {
int originalNumber = number;
int sum = 0;
int numberOfDigits = String.valueOf(number).length();
while (number != 0) {
int digit = number % 10;
sum += Math.pow(digit, numberOfDigits);
number /= 10;
}
return sum == originalNumber;
}
}
Explanation of the Code
Let’s break down the program step-by-step to understand how it works.
- Importing the Scanner Class: We import the
Scanner
class from thejava.util
package to read input from the user.
import java.util.Scanner;
2. Creating the Main Class: We create a public class named ArmstrongNumber
. In Java, the class name should match the filename.
public class ArmstrongNumber {
3. Main Method: The main
method is the entry point of the program. Inside this method, we will write the code to check if a number is an Armstrong number.
public static void main(String[] args) {
4. Creating a Scanner Object: We create a Scanner
object to read input from the user.
Scanner scanner = new Scanner(System.in);
5. Reading Input from the User: We prompt the user to enter a number and store this value in a variable.
System.out.print("Enter a number: ");
int number = scanner.nextInt();
6. Checking if the Number is an Armstrong Number: We call the isArmstrongNumber
method to check if the number is an Armstrong number. The result is stored in a variable isArmstrong
.
boolean isArmstrong = isArmstrongNumber(number);
7. Printing the Result: We print whether the number is an Armstrong number or not based on the result.
if (isArmstrong) {
System.out.println(number + " is an Armstrong number.");
} else {
System.out.println(number + " is not an Armstrong number.");
}
8. Method to Check if a Number is an Armstrong Number: The isArmstrongNumber
method checks if the given number is an Armstrong number. This method works by calculating the sum of the digits each raised to the power of the number of digits and comparing the sum to the original number.
public static boolean isArmstrongNumber(int number) {
int originalNumber = number;
int sum = 0;
int numberOfDigits = String.valueOf(number).length();
while (number != 0) {
int digit = number % 10;
sum += Math.pow(digit, numberOfDigits);
number /= 10;
}
return sum == originalNumber;
}
Java program to check if a number is an Armstrong number
Top 100 Java Programs: Click Here
Are you looking for a job: Click Here
Explanation of the isArmstrongNumber
Method
- Initializing Variables: We initialize
originalNumber
to store the original number,sum
to store the sum of the digits raised to the power of the number of digits, andnumberOfDigits
to store the number of digits in the original number.
int originalNumber = number;
int sum = 0;
int numberOfDigits = String.valueOf(number).length();
2. Loop to Calculate the Sum: The while
loop continues as long as number
is not zero. In each iteration, we extract the last digit of number
using number % 10
, raise it to the power of numberOfDigits
, add it to sum
, and then remove the last digit from number
by dividing it by 10.
while (number != 0) {
int digit = number % 10;
sum += Math.pow(digit, numberOfDigits);
number /= 10;
}
3. Returning the Result: After the loop finishes, we return true
if sum
is equal to originalNumber
, otherwise, we return false
.
return sum == originalNumber;
Running the Program
To run the program, follow these steps:
- Save the code in a file named
ArmstrongNumber.java
. - Open a command prompt or terminal and navigate to the directory where you saved the file.
- Compile the program using the following command:
javac ArmstrongNumber.java
4. Run the compiled program using the following command:
java ArmstrongNumber
5. Follow the prompt to enter a number. The program will display whether the number is an Armstrong number or not.
Example Output
If you run the program and enter 153 as the number, the output will be:
Enter a number: 153
153 is an Armstrong number.
If you run the program and enter 123 as the number, the output will be:
Enter a number: 123
123 is not an Armstrong number.
What did we learn from this article?
Checking if a number is an Armstrong number is a fundamental exercise that helps in understanding loops, arithmetic operations, and the concept of number manipulation in programming. This Java program demonstrates how to check if a number is an Armstrong number by calculating the sum of its digits each raised to the power of the number of digits and comparing the sum to the original number. By practicing such basic programs, you can strengthen your problem-solving skills and gain confidence in your ability to write efficient Java code. Keep practicing and exploring more problems to enhance your programming skills.