Table of Contents
Java program to print the Fibonacci series- The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. The sequence commonly starts as 0, 1, 1, 2, 3, 5, 8, and so on. Printing the Fibonacci series up to n
terms is a common exercise in programming to understand loops and sequences.
Java Program to Print Fibonacci Series up to n
Terms
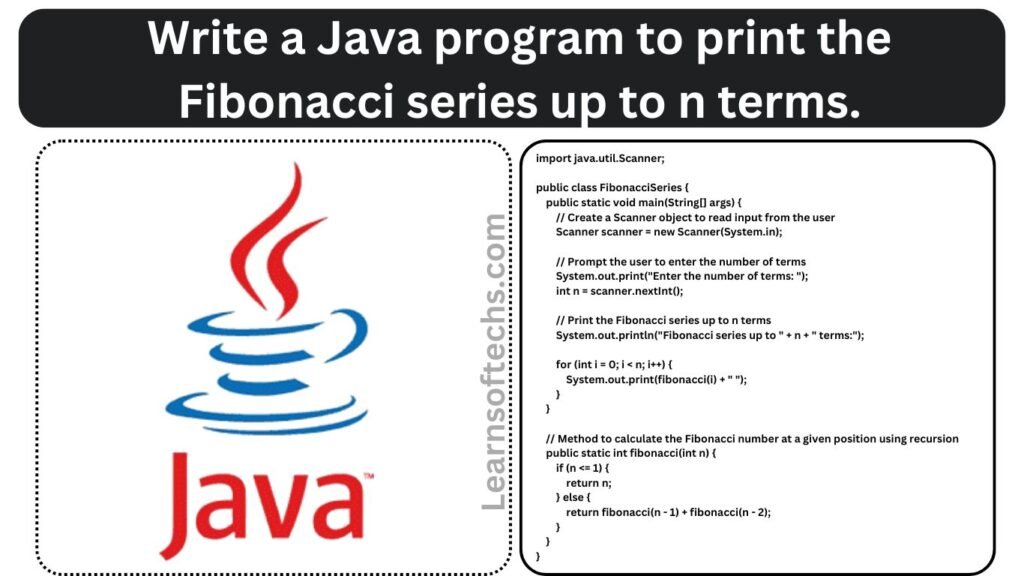
Here’s a Java program that prompts the user to enter the number of terms n
and then prints the Fibonacci series up to n
terms.
Java program to print the Fibonacci series
import java.util.Scanner;
public class FibonacciSeries {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter the number of terms
System.out.print("Enter the number of terms: ");
int n = scanner.nextInt();
// Print the Fibonacci series up to n terms
System.out.println("Fibonacci series up to " + n + " terms:");
for (int i = 0; i < n; i++) {
System.out.print(fibonacci(i) + " ");
}
}
// Method to calculate the Fibonacci number at a given position using recursion
public static int fibonacci(int n) {
if (n <= 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
}
Explanation of the Code:- Java program to print the Fibonacci series
Let’s break down the program step-by-step to understand how it works.
- Importing the Scanner Class: We import the
Scanner
class from thejava.util
package to read input from the user.
import java.util.Scanner;
2. Creating the Main Class: We create a public class named FibonacciSeries
. In Java, the class name should match the filename.
public class FibonacciSeries {
3. Main Method: The main
method is the entry point of the program. Inside this method, we will write the code to print the Fibonacci series.
public static void main(String[] args) {
4. Creating a Scanner Object: We create a Scanner
object to read input from the user.
Scanner scanner = new Scanner(System.in);
5. Reading Input from the User: We prompt the user to enter the number of terms n
and store this value in a variable.
System.out.print("Enter the number of terms: ");
int n = scanner.nextInt();
6. Printing the Fibonacci Series: We use a for
loop to print the Fibonacci series up to n
terms. The fibonacci
method is called for each term to calculate its value.
System.out.println("Fibonacci series up to " + n + " terms:");
for (int i = 0; i < n; i++) {
System.out.print(fibonacci(i) + " ");
}
7. Method to Calculate Fibonacci Number: The fibonacci
method calculates the Fibonacci number at a given position using recursion. If the position n
is 0 or 1, it returns n
. Otherwise, it returns the sum of the Fibonacci numbers at positions n-1
and n-2
.
public static int fibonacci(int n) {
if (n <= 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
Running the Program
To run the program, follow these steps:
- Save the code in a file named
FibonacciSeries.java
. - Open a command prompt or terminal and navigate to the directory where you saved the file.
- Compile the program using the following command:
javac FibonacciSeries.java
4. Run the compiled program using the following command:
java FibonacciSeries
5. Follow the prompt to enter the number of terms. The program will display the Fibonacci series up to the specified number of terms.
Example Output:- Java program to print the Fibonacci series
If you run the program and enter 10 as the number of terms, the output will be:
Enter the number of terms: 10
Fibonacci series up to 10 terms:
0 1 1 2 3 5 8 13 21 34
Top 100 Java Programs: Click Here
Are you looking for a job: Click Here
What did you learn from this article?
Printing the Fibonacci series up to n
terms is a fundamental exercise that helps in understanding recursion and sequences in programming. This Java program demonstrates how to implement the Fibonacci series using a recursive method. By practicing such basic programs, you can strengthen your problem-solving skills and gain confidence in your ability to write efficient Java code. Keep practicing and exploring more problems to enhance your programming skills.