Table of Contents
Java program to print the multiplication table of a number. Printing a multiplication table of a given number is a straightforward programming task that involves looping through a range of numbers and multiplying each by the given number. Here’s a Java program that prompts the user to enter a number and then prints its multiplication table up to 10.
Java Program to Print the Multiplication Table of a Number
import java.util.Scanner;
public class MultiplicationTable {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter a number
System.out.print("Enter a number: ");
int number = scanner.nextInt();
// Print the multiplication table
printMultiplicationTable(number);
}
// Method to print the multiplication table of a number
public static void printMultiplicationTable(int n) {
for (int i = 1; i <= 10; i++) {
int result = n * i;
System.out.println(n + " x " + i + " = " + result);
}
}
}
Explanation of the Code
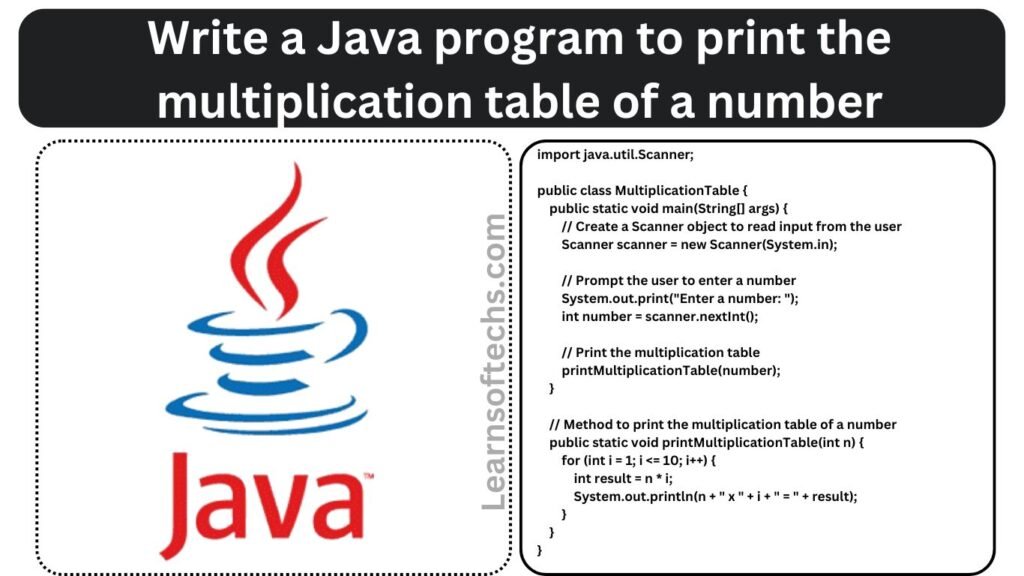
Let’s break down the program step-by-step to understand how it works.
- Importing the Scanner Class: We import the
Scanner
class from thejava.util
package to read input from the user.
import java.util.Scanner;
2. Creating the Main Class: We create a public class named MultiplicationTable
. In Java, the class name should match the filename.
public class MultiplicationTable {
3. Main Method: The main
method is the entry point of the program. Inside this method, we will write the code to print the multiplication table of a number.
public static void main(String[] args) {
4. Creating a Scanner Object: We create a Scanner
object to read input from the user.
Scanner scanner = new Scanner(System.in);
5. Reading Input from the User: We prompt the user to enter a number and store this value in a variable.
System.out.print("Enter a number: ");
int number = scanner.nextInt();
6. Printing the Multiplication Table: We call the printMultiplicationTable
method to print the multiplication table of the number.
printMultiplicationTable(number);
7. Method to Print the Multiplication Table: The printMultiplicationTable
method prints the multiplication table of the given number from 1 to 10. This method uses a for
loop to iterate through the numbers from 1 to 10, multiplying each by the given number and printing the result.
public static void printMultiplicationTable(int n) {
for (int i = 1; i <= 10; i++) {
int result = n * i;
System.out.println(n + " x " + i + " = " + result);
}
}
Explanation of the printMultiplicationTable
Method
- Loop to Print the Multiplication Table: The
for
loop iterates through the numbers from 1 to 10. In each iteration, it multiplies the given numbern
by the loop variablei
and prints the result in the formatn x i = result
.
for (int i = 1; i <= 10; i++) {
int result = n * i;
System.out.println(n + " x " + i + " = " + result);
}
Running the Program
To run the program, follow these steps:
- Save the code in a file named
MultiplicationTable.java
. - Open a command prompt or terminal and navigate to the directory where you saved the file.
- Compile the program using the following command:
javac MultiplicationTable.java
4. Run the compiled program using the following command:
java MultiplicationTable
5. Follow the prompt to enter a number. The program will display its multiplication table.
Example Output
If you run the program and enter 5 as the number, the output will be:
Enter a number: 5
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
If you run the program and enter 7 as the number, the output will be:
Enter a number: 7
7 x 1 = 7
7 x 2 = 14
7 x 3 = 21
7 x 4 = 28
7 x 5 = 35
7 x 6 = 42
7 x 7 = 49
7 x 8 = 56
7 x 9 = 63
7 x 10 = 70
Top 100 Java Programs: Click Here
Are you looking for a job: Click Here
What did we learn from this article?
Printing the multiplication table of a number is a fundamental exercise that helps in understanding loops and arithmetic operations in programming. This Java program demonstrates how to print the multiplication table of a number by iterating through a range of numbers and multiplying each by the given number. By practicing such basic programs, you can strengthen your problem-solving skills and gain confidence in your ability to write efficient Java code. Keep practicing and exploring more problems to enhance your programming skills.